Python - #5 - Selection
1. Using if Statements
Using if enables your program to make a choice.
​
There are a few things you need to remember:
-
if is lowercase - it should turn orange.
-
You must use double equals ==
-
You need a colon : at the end of your if line.
-
The line below your if line must be indented.
​


Task 1 - Create a new Python program and save the file as 5-Selection.py
​
Use the picture to help you ask what your favourite food is. Run the program and test it works.
To indent a line press the tab key
on your keyboard.
​
Indentation is important as it tells Python what is within the if statement and what isn't.
2. Using elif
elif stands for 'else if'. It is used to respond in a different way depending on the input.
​
elif works exactly the same as an if line so if you make a mistake look up at task 1 to help you.
Task 2 - Write an elif line that responds differently to your favourite food question from task 1. e.g. "Yum!" if someone enters "pasta".

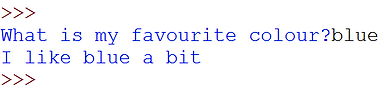
3. Using else
It is impractical to have hundreds of elif lines to respond to different inputs.
​
else is used to respond to anything else that has been entered in a general way.
​
The else line works a bit differently, so look carefully at the picture.

Task 3 - Write an else line that responds to anything else the user enters for your favourite food question.
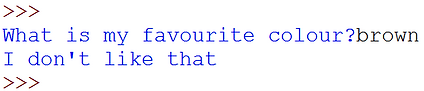
4. Multiple elifs
Despite what you did in task 3, programs can be expanded with more than one elif line.
​
Underneath your first elif line but before your else line, add at least two more elif sections that respond differently depending on what is entered.
​
Use the elif line from the task 2 picture to help you.
Task 4 - Read the description above and use task 2 to help you.

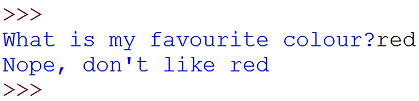
Challenge Programs
Use everything that you have learned on this page to help you create these programs...
​
Challenge Task 1 - Spanish Translation
-
Create a new Python program. Save it as '5-Translator.py'
-
Add a comment at the top with your name and the date.
-
Create a program that asks for a number between 1 and 4.
-
Use if and elif statements to see what the user has entered and print a statement that displays the chosen number in Spanish - use the image to help you understand.
​
-
BONUS: Add an else line for any numbers higher than 4.
​​
When you run it, it could look something like this:

Challenge Task 2 - Able to Vote
-
Create a new Python program. Save it as '5-Vote.py'
-
Add a comment at the top with your name and the date.
-
Create a program that asks for their age.
-
Use an if statement to see if the age is more than 17 (use > instead of ==).
-
If the age is over 17, then print "You are old enough to vote!"
-
Use an else statement to print a different message for everyone else.
​​
When you run it, it could look something like this:
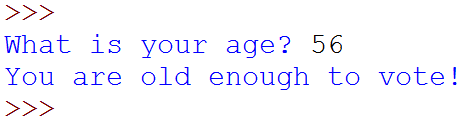
Challenge Task 3 - Totals
-
Create a new Python program. Save it as '5-Totals.py'
-
Add a comment at the top with your name and the date.
-
Use an int input line to ask the user for number 1.
-
Use an int input line to ask the user for number 2.
-
Multiply the two numbers together and save it into a variable called total.
-
If the total is over 9000, then print "It's over 9,000!!!"
-
Use an else statement to print the total if it is less than 9000.
​​
When you run it, it could look something like this:
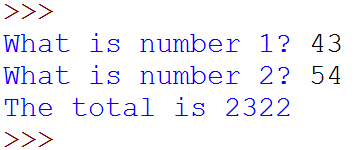
